Sprint 0: Getting Started¶
First (Early Bonus) Deadline: Jan 17 at 11:59pm
Second Deadline: Jan 22 at 11:59pm
Objective¶
This is an individual assignment to get everyone comfortable using Visual Studio and/or VSCode, .NET, C#, and MonoGame, as well as designing and implementing objects and interfaces. Advanced students may also make use of the Command design pattern. The core requirements of this Sprint are content loading, sprite drawing, and input handling, in an event loop.
This assignment has two due dates. You are expected to turn in whatever you have completed by the first deadline, but will be allowed to turn in your work again without a late penalty as long as the resubmission is turned in by the second deadline. If you completly finish the assignment by the first deadline you will earn a 5% extra credit bonus, possibly going over 100% for the assignment.
Sprint Outline¶
Step 1: Software Setup¶
The short version of this step is to follow MonoGame’s Getting Started Page and ensure you can create, build, and run an “empty” MonoGame project. If successful, you should have an application that shows a blue window when it runs. You can proceed to step 2 once you have this accomplished. The rest of this section provides more detailed information on environment setup and troubleshooting.
It is recommended that you use a device running Windows for this course. There is a native option for Macs, but we cannot help with troubleshooting them as much. Virtual machines are an option but tend to run much slower than you’d prefer, so using a second partition for Windows is recommended if you don’t have a Windows PC already. Machines in CL112 may or may not have the most up to date versions of the software we are using. We’ll be using Visual Studio 2022 and Monogame version 3.8.2.
You can proceed right to MonoGame’s Getting Started Page page as it now includes steps on installing Visual Studio and VSCode with the necessary modules/plug-ins. If using Visual Studio, the (free) Community edition is just fine for this course.
Miscellaneous Troubleshooting - read these before you ask for help on the install!
The most common of unexpected problems encountered here stem from Visual Studio behaving poorly when dealing with white space in file names when building MonoGame projects, the first reported error is usually: “…Xna namespace cannot be found…” when this occurs. Make sure the Visual Studio project you create does not contain whitespace characters to avoid this problem. This may no longer be an issue with recent Visual Studio versions, but it cannot be ruled out. In short, when you name your project don’t include whitespace, and place your project in a path without spaces.
To use the MGCB Editor tool for building of content projects, you may need to install some Visual C++ Redistributables. If your development machine is one you also use for gaming you may already have some of these installed without realizing it. Based on setup in previous semesters, we recommend downloading and running the 2012, 2013, and 2019 packages. The most common error message that should cue you that you need to do these steps is “Unable to build content - Cannot load FreeImage”; more info on this error can be found here and here.
For students working out of Caldwell Labs, the project templates may not be showing up (we had this issue with MonoGame 3.7.1 but it might not reproduce with 3.8). If that is the case, either create the project using the CLI (see here) or contact me and I’ll create and post an instance of an empty starter project for you to use to get started.
Also for students working out of Caldwell Labs, note that Visual Studio is used, not Visual Studio Blend.
The MonoGame community isn’t incredibly large, but you might find additional install and setup help on their forums.
Step 2: Working with MonoGame - Basic Tutorials¶
For understanding the basics of working with MonoGame, there are two sets of tutorials you might find useful. It is suggested that you work through these tutorials to get a better understanding of MonoGame, and specifically the parts of it which we will use in this course.
The first are the three official examples listed in the MonoGame’s Getting Started Page under sections 1 through 5. These cover what the starter code does, how to load images into the project using the MonoGame Content Builder Tool (MGCB Editor), and writing code that draws images.
The second are the Legacy Tutorials - these use terms and have screenshots from an older version of MonoGame, but include an example of more reusable code for sprite drawing that we’ll look at in class. The MonoGame concepts and APIs they introduce are still relevant for current MonoGame versions.
Step 3: Programming Assignment¶
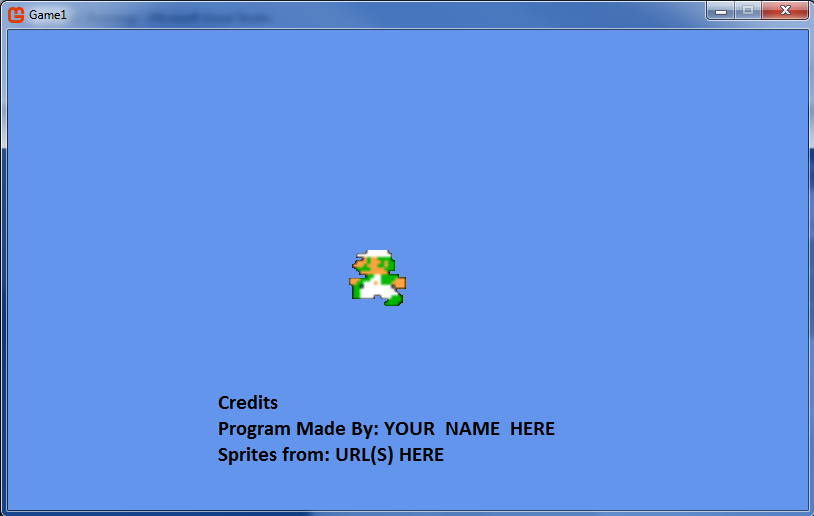
Your task is to implement a very simple interactive program. At run-time, the
user should be able to select between display of a motionless and non-animated
sprite (ex: luigi standing still), a motionless and animated sprite (ex: luigi
running in place), a moving and non-animated sprite (ex: dead luigi floating up
and down), and a moving and animated sprite (ex: luigi running right and left).
Note: only one of these sprites should be visible at a time. The user should
also be able to quit the program with a key press (the 0
key). Additionally,
you should use a text sprite to display your name and the source of your art
assets. One significant purpose of this assignment is to gain experience using
interfaces (designing them, implementing them, and using them like objects).
Carefully read and follow these requirements:
Create some interfaces:
Create an interface for any and all controllers,
IController
.Create an interface for any and all sprites,
ISprite
.Optionally, using the Command Design Pattern, create an ICommand interface.
Then make some concrete classes that implement the interfaces:
Implement a concrete class for a Keyboard controller.
Implement a concrete class for a Mouse controller.
Implement a concrete class for the non-moving, non-animated sprite.
Implement a concrete class for the non-moving, animated sprite.
Implement a concrete class for the moving, non-animated sprite.
Implement a concrete class for the moving, animated sprite.
Implement a concrete class for a text sprite.
If you made an
ICommand
interface, implement a concrete class for each Command the user can trigger: one for each different sprite that can be set and one to quit the game.
For keyboard input:
Have a key (0) to quit.
Have a key (1) that has the program display a sprite with only one frame of animation and a fixed position. This should be the initial state of the program.
Have a key (2) that has the program display an animated sprite, but with a fixed position.
Have a key (3) that has the program display a sprite with only one frame of animation, but moves the sprite up and down on screen.
Have a key (4) that has the program display an animated sprite, moving to the left and right on screen.
Note on sprite motion: Instead of the moving sprites periodically changing direction, you may opt to have the sprite move in only one direction and have its position wrap around to the opposite edge once it reaches the end of the screen.
For mouse input:
Left mouse click, when the mouse cursor is in the top left quarter of the window, should display a sprite with only one frame of animation and a fixed position (i.e. the same as pressing 1).
Left mouse click, when the mouse cursor is in the top right quarter of the window, should display an animated sprite, but with a fixed position (i.e. key 2).
Left mouse click, when the mouse cursor is in the bottom left quarter of the window, should display a sprite with only one frame of animation, but moves the sprite up and down on screen (i.e. key 3).
Left mouse click, when the mouse cursor is in the bottom right quarter of the window, should display an animated sprite, moving to the left and right on screen (i.e. key 4).
A visual example of these four portions, labeled as Quad#, is shown below.
Right-mouse click should quit.
Finally, in the main game class, use the interfaces to animate/update the current sprite. If you are not using commands you will also need logic to switch between sprites and quit in the main game class (or in the concrete controllers). IMPORTANT: Your main game class should only refer to the sprites and controllers through the
ISprite
andIController
interfaces, respectively, except for the initial creation of the objects.
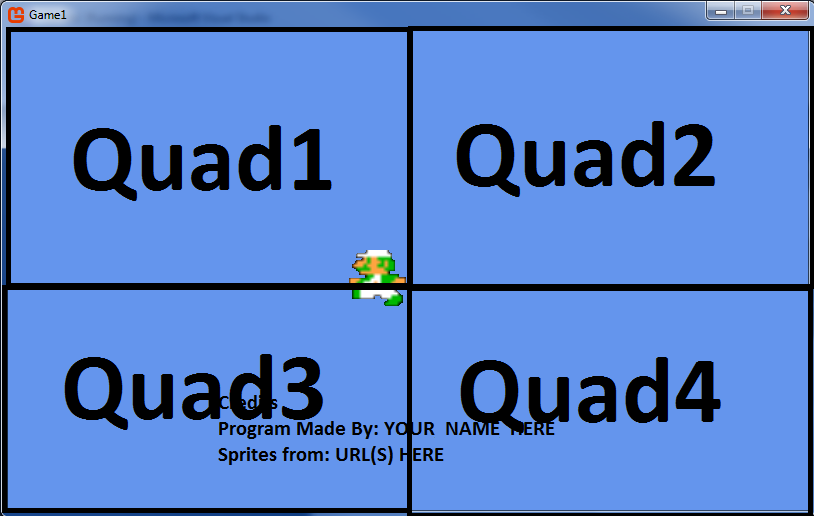
The above is an illustration of what is meant by quad. You don’t have to draw the lines or Quad# text in the actual program.
Design suggestions:
Include a
Dictionary
in the Keyboard controller class that maps keys (buttons) to concrete instances ofICommand
(based on the input keys/buttons listed above).In the
Update
method of the controllers, for each input key/button that is pressed, create and execute its corresponding Command.Alternatively, for the controller code if you are not using Commands, consider splitting up input logic into multiple classes (in this case
QuittingController
andSpriteSettingController
).In the
Game
(main) class, only use the interfaces in the Update and Draw methods.
Sprite Resources:
You can find spritesheets for Super Mario Bros. and The Legend of Zelda off of links on the Resources page.
You are free to use other spritesheets, but do not use a spritesheet that is already is the format of a texture atlas - the learning objective here is in figuring out how to work with any spritesheet, not just ‘convenient’ ones. You may use a spritesheet formatted as a texture atlas if you edit the image to have this format yourself - include a before and after image in your submission if you do this.
IMPORTANT: Do NOT use the smiley walk animation from the above tutorials or copy/paste the sprite animation code from the tutorials. The spritesheet is already formatted as a texture atlas. Your sprite animation code must be your own!
Project Template:
If you are having difficulty installing the MonoGame templates and creating an initial project, you can use the CSE3902 Group Project Template. But please try to create your own project first as it is a valuable learning experience.
Work to turn in:
Do a Build->Clean in Visual Studio, and then without rebuilding, zip/compress the directory containing your solution.
It is recommended that you unzip the file elsewhere, then build and run it to see if it is working correctly.
Turn in zipped file on Carmen under the assignment page named “Sprint0”.
The dropbox is set up to accept multiple submissions, only the latest submission before the deadline will be graded.
Start early and do not wait until the last minute to submit work! Seriously, this is the number one issue with students not completing the assignment!
Grading Criteria¶
If you cannot complete enough functionality to reach 60% on this assignment, you will have a difficult time being able to contribute on the team project. You should be able to evaluate your work using the rubric provided below and reach out for help before the assignment’s deadline if it looks like you will come up short. If you are not able to complete the assignment by the date of the resubmission option it is recommended that you consider dropping the course. Understanding the basics of sprite animation and user input is a fundamental building block of this course. If you have any questions, ASK!
Your grade on this assignment will be based on correctness, but you will also receive feedback on how you could improve upon the quality of your code.
Correctness (does it work)
Support for keyboard input |
20% |
Support for mouse input |
20% |
Support for the four sprites types |
10% each; 40% total |
Support for text drawing for the application’s credits |
20% |
Code quality (is the code simple, readable, maintainable, and reusable)
Readability: Follow C# capitalization conventions, as shown here. To summarize:
Most names will be in PascalCase where the first letter is capitalized along with the start of each word concatenated to it.
The exceptions where camelCase (first letter lowercase then capitalize the start of concatenated words) is used for parameterNames and classMembers
You do not need to use ‘_’ for private members.
Readability: Use meaningful names for interfaces, classes, and variables so that you do not need or have any comments explaining them
Maintainability: The interfaces should be complete, but as simple as possible
Maintainability: Using interfaces in the Game class instead of the concrete types
Simplicity and Reusability: Following the design suggestions in the list of objects and interfaces above (if you want to come up with your own design talk to the instructor first)